
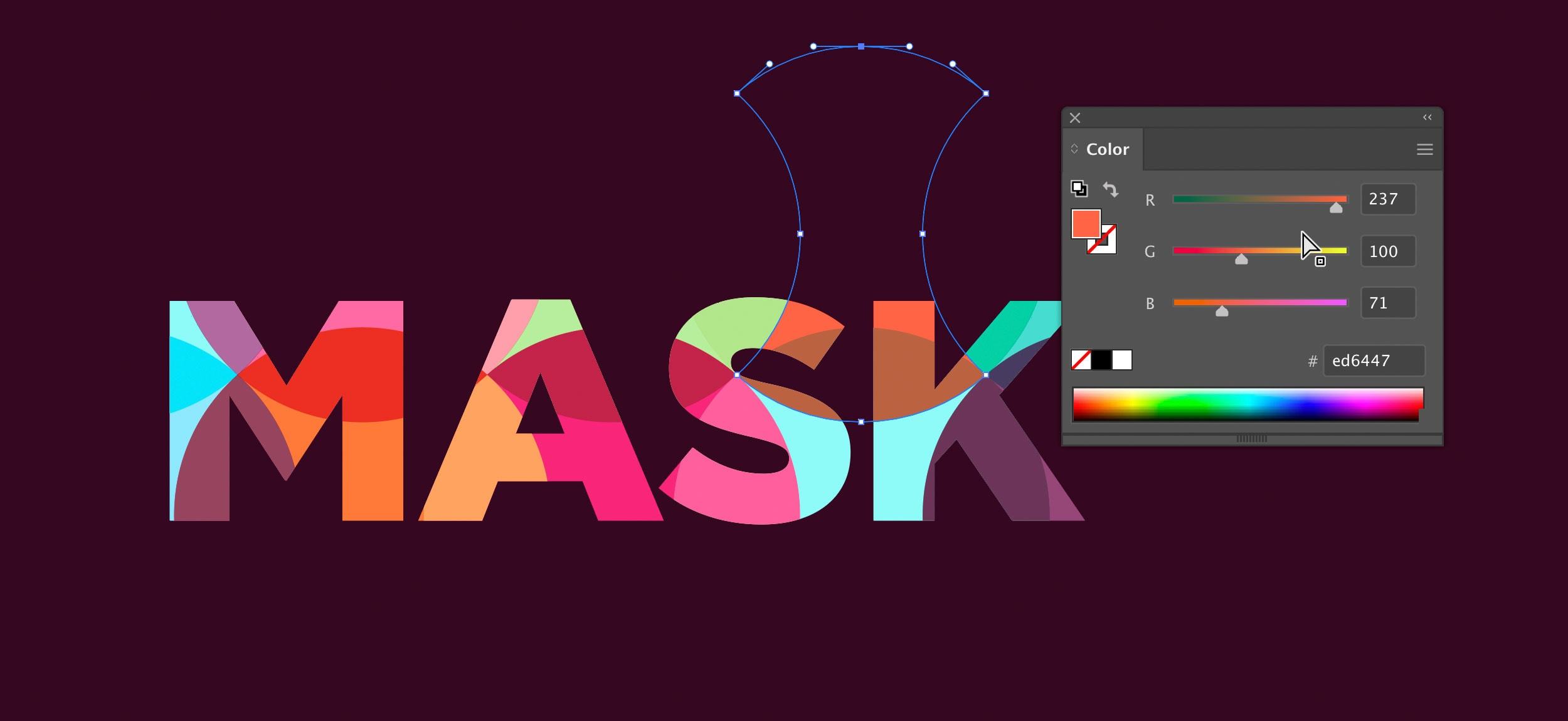
# Use the decorator to make `outer_function` a outer_function(x): Tf.function applies to a function and all other functions it calls: def inner_function(x, y, b): That is how a Function is able to give you the benefits of graph execution, like speed and deployability (refer to The benefits of graphs above). A Function encapsulates several tf.Graphs behind one API (learn more in the Polymorphism section). Underneath, however, it is very different.

On the outside, a Function looks like a regular function you write using TensorFlow operations. Tf_function_value = a_function_that_uses_a_graph(x1, y1, b1).numpy() # Call a `Function` like a Python function. Orig_value = a_regular_function(x1, y1, b1).numpy() # `a_function_that_uses_a_graph` is a TensorFlow `Function`.Ī_function_that_uses_a_graph = tf.function(a_regular_function) You use a Function in the same way as its Python equivalent. A Function is a Python callable that builds TensorFlow graphs from the Python function. tf.function takes a regular function as input and returns a Function. You create and run a graph in TensorFlow by using tf.function, either as a direct call or as a decorator. Import some necessary libraries: import tensorflow as tf However, you still want to define your machine learning models (or other computations) in Python for convenience, and then automatically construct graphs when you need them. In short, graphs are extremely useful and let your TensorFlow run fast, run in parallel, and run efficiently on multiple devices. There is an entire optimization system, Grappler, to perform this and other speedups. Simplify arithmetic operations by eliminating common subexpressions.Separate sub-parts of a computation that are independent and split them between threads or devices.Statically infer the value of tensors by folding constant nodes in your computation ("constant folding").Graphs are also easily optimized, allowing the compiler to do transformations like: TensorFlow uses graphs as the format for saved models when it exports them from Python. You can use your TensorFlow graph in environments that don't have a Python interpreter, like mobile applications, embedded devices, and backend servers. With a graph, you have a great deal of flexibility. This is what a TensorFlow graph representing a two-layer neural network looks like when visualized in TensorBoard: Since these graphs are data structures, they can be saved, run, and restored all without the original Python code. Graphs are data structures that contain a set of tf.Operation objects, which represent units of computation and tf.Tensor objects, which represent the units of data that flow between operations. Graph execution means that tensor computations are executed as a TensorFlow graph, sometimes referred to as a tf.Graph or simply a "graph." While eager execution has several unique advantages, graph execution enables portability outside Python and tends to offer better performance. This means TensorFlow operations are executed by Python, operation by operation, and returning results back to Python. In the previous three guides, you ran TensorFlow eagerly. For a more complete specification of tf.function, go to the Better performance with tf.function guide. This is a big-picture overview that covers how tf.function allows you to switch from eager execution to graph execution. Note: For those of you who are only familiar with TensorFlow 1.x, this guide demonstrates a very different view of graphs.
#Keyshape wrapping object around another object masking code#
In this guide, you'll learn how TensorFlow allows you to make simple changes to your code to get graphs, how graphs are stored and represented, and how you can use them to accelerate your models. If you instead want to immediately get started with Keras, check out the collection of Keras guides. This guide goes beneath the surface of TensorFlow and Keras to demonstrate how TensorFlow works.
